6DOF Robotics Arm Documentation
A 6DOF (Six Degrees of Freedom) robotic arm is a type of robotic arm that can move in six different ways, providing a high level of flexibility and precision. The “degrees of freedom” refer to the independent movements it can make, typically in three rotational axes (pitch, yaw, and roll) and three translational axes (movement along the X, Y, and Z axes).
- Base Rotation (Yaw): This allows the base of the arm to rotate, providing the ability to move in the horizontal plane.
- Shoulder Movement (Pitch): This controls the arm’s upward and downward movement.
- Elbow Movement (Pitch): This moves the forearm closer to or farther from the body of the arm.
- Wrist Pitch: This moves the wrist up and down.
- Wrist Roll: This rotates the wrist around its own axis.
- Wrist Yaw: This rotates the wrist side to side, allowing the end effector (e.g., gripper or tool) to adjust orientation.
Reference Material
Servo Motor Interfacing with Arduino Uno

Program:
#include <Servo.h>
Servo myservo;
void setup()
{
myservo.attach(9);
}
void loop() {
myservo.write(180);
}
Bluetooth interfacing with Arduino
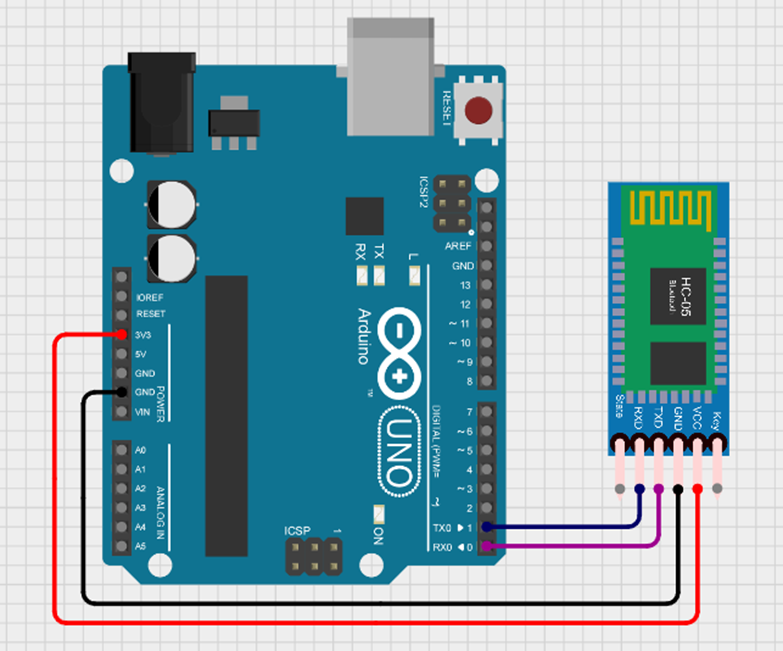
Program:
char cmd;
void setup() {
Serial.begin(9600);
}
void loop() {
if(Serial.available()>0){
cmd = Serial.read();
Serial.println(cmd);
}
}
Bluetooth Application for controlling Robot Arm
Android App Link – download app
ios App Link – download app
Servo Motor & Bluetooth interfacing with Arduino Uno

Program:
#include<Servo.h>
Servo gate;
char cmd;
void setup() {
Serial.begin(9600);
gate.attach(9);
}
void loop() {
if(Serial.available()>0){
cmd = Serial.read();
Serial.println(cmd);
if(cmd=='B'){
gate.write(90);
}
if(cmd=='b'){
gate.write(0);
}
}
}
Final Ble Control Robotic Arm Circuit Diagram
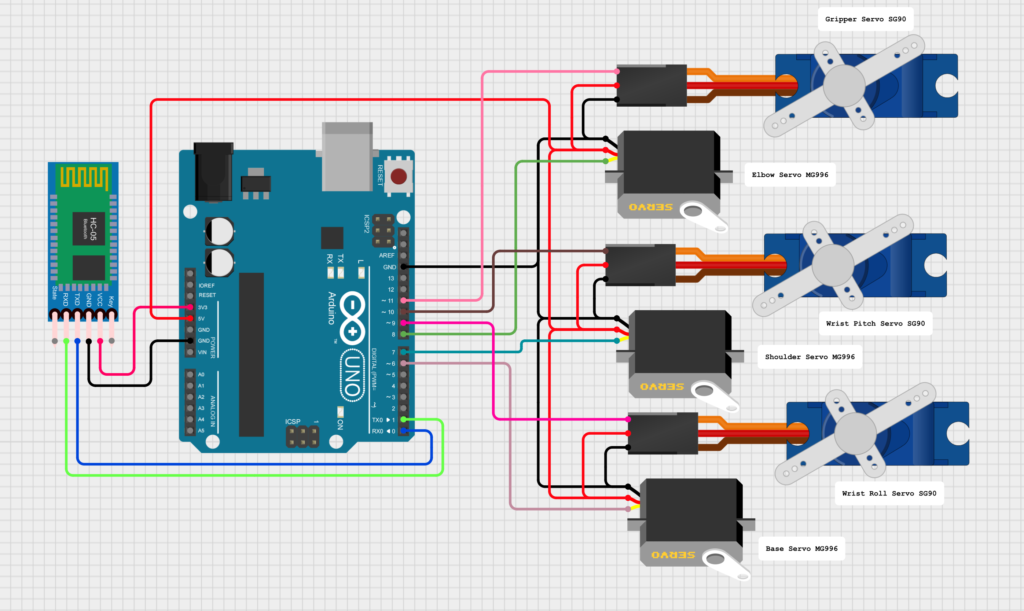
Final Ble Control Robotic Arm Code
#include <Servo.h>
// Define Servo objects
Servo baseServo, shoulderServo, elbowServo, wristRollServo, wristPitchServo, gripperServo;
// Define pins and initial angles
const int basePin = 6, shoulderPin = 7, elbowPin = 8, wristRollPin = 9, wristPitchPin = 10, gripperPin = 11;
int baseAngle = 90, shoulderAngle = 90, elbowAngle = 90, wristRollAngle = 90, wristPitchAngle = 90, gripperAngle = 95;
// Step delay for smooth motion
const int stepDelay = 20;
// Function to move servo smoothly
void moveServo(Servo &servo, int ¤tAngle, int targetAngle) {
if (currentAngle < targetAngle) {
for (int angle = currentAngle; angle <= targetAngle; angle++) {
servo.write(angle);
delay(stepDelay);
}
} else {
for (int angle = currentAngle; angle >= targetAngle; angle--) {
servo.write(angle);
delay(stepDelay);
}
}
currentAngle = targetAngle;
}
void setup() {
// Attach all servos and set initial positions
baseServo.attach(basePin);
baseServo.write(baseAngle);
shoulderServo.attach(shoulderPin);
shoulderServo.write(shoulderAngle);
elbowServo.attach(elbowPin);
elbowServo.write(elbowAngle);
wristRollServo.attach(wristRollPin);
wristRollServo.write(wristRollAngle);
wristPitchServo.attach(wristPitchPin);
wristPitchServo.write(wristPitchAngle);
gripperServo.attach(gripperPin);
gripperServo.write(gripperAngle);
// Initialize serial communication
Serial.begin(9600);
}
void loop() {
if (Serial.available()) {
char command = Serial.read(); // Read the received command
Serial.println(command); // Debugging: print received command
// Control each servo based on the command
switch (command) {
case 'B': // Base Clockwise
moveServo(baseServo, baseAngle, min(baseAngle + 10, 180));
break;
case 'b': // Base Anticlockwise
moveServo(baseServo, baseAngle, max(baseAngle - 10, 0));
break;
case 'S': // Shoulder Clockwise
moveServo(shoulderServo, shoulderAngle, min(shoulderAngle + 5, 120));
break;
case 's': // Shoulder Anticlockwise
moveServo(shoulderServo, shoulderAngle, max(shoulderAngle - 5, 60));
break;
case 'E': // Elbow Clockwise
moveServo(elbowServo, elbowAngle, min(elbowAngle + 10, 180));
break;
case 'e': // Elbow Anticlockwise
moveServo(elbowServo, elbowAngle, max(elbowAngle - 10, 0));
break;
case 'R': // Wrist Roll Clockwise
moveServo(wristRollServo, wristRollAngle, min(wristRollAngle + 10, 180));
break;
case 'r': // Wrist Roll Anticlockwise
moveServo(wristRollServo, wristRollAngle, max(wristRollAngle - 10, 0));
break;
case 'W': // Wrist Pitch Clockwise
moveServo(wristPitchServo, wristPitchAngle, min(wristPitchAngle + 10, 180));
break;
case 'w': // Wrist Pitch Anticlockwise
moveServo(wristPitchServo, wristPitchAngle, max(wristPitchAngle - 10, 0));
break;
case 'G': // Gripper Open
moveServo(gripperServo, gripperAngle, min(gripperAngle + 5, 180));
break;
case 'g': // Gripper Close
moveServo(gripperServo, gripperAngle, max(gripperAngle - 5, 95));
break;
default:
Serial.println("Invalid Command");
break;
}
}
}
Pick & Place Robot Code:
#include <Servo.h>
// Define Servo objects
Servo baseServo, shoulderServo, elbowServo, wristRollServo, wristPitchServo, gripperServo;
// Define pins and initial angles
const int basePin = 6, shoulderPin = 7, elbowPin = 8, wristRollPin = 9, wristPitchPin = 10, gripperPin = 11;
int baseAngle = 90, shoulderAngle = 90, elbowAngle = 90, wristRollAngle = 90, wristPitchAngle = 90, gripperAngle = 95;
// Step delay for smooth motion
const int stepDelay = 20;
// Function to move servo smoothly
void moveServo(Servo &servo, int ¤tAngle, int targetAngle) {
if (currentAngle < targetAngle) {
for (int angle = currentAngle; angle <= targetAngle; angle++) {
servo.write(angle);
delay(stepDelay);
}
} else {
for (int angle = currentAngle; angle >= targetAngle; angle--) {
servo.write(angle);
delay(stepDelay);
}
}
currentAngle = targetAngle;
}
void setup() {
// Attach all servos and set initial positions
baseServo.attach(basePin);
baseServo.write(baseAngle);
shoulderServo.attach(shoulderPin);
shoulderServo.write(shoulderAngle);
elbowServo.attach(elbowPin);
elbowServo.write(elbowAngle);
wristRollServo.attach(wristRollPin);
wristRollServo.write(wristRollAngle);
wristPitchServo.attach(wristPitchPin);
wristPitchServo.write(wristPitchAngle);
gripperServo.attach(gripperPin);
gripperServo.write(gripperAngle);
}
void loop() {
// No operation in loop as movements are delay-based
// Pick operation
delay(3000);
moveServo(baseServo, baseAngle, 100); // Move base to pick position
moveServo(shoulderServo, shoulderAngle, 120); // Lower shoulder
moveServo(elbowServo, elbowAngle, 40); // Extend elbow
moveServo(gripperServo, gripperAngle, 95); // Close gripper (Pick object)
delay(1000);
moveServo(shoulderServo, shoulderAngle, 90); // Lift object
moveServo(elbowServo, elbowAngle, 60);
// Place operation
delay(3000);
moveServo(baseServo, baseAngle, 160); // Move base to place position
moveServo(shoulderServo, shoulderAngle, 120); // Lower shoulder
moveServo(elbowServo, elbowAngle, 40); // Extend elbow
moveServo(gripperServo, gripperAngle, 180); // Open gripper (Release object)
delay(1000);
moveServo(shoulderServo, shoulderAngle, 90); // Return to home position
moveServo(elbowServo, elbowAngle, 60);
}
Applications:
- Industrial Automation: Manufacturing tasks like assembly, welding, and packaging.
- Research and Development: Prototyping new robotic movements or advanced control algorithms.
- Medical Field: Assisting in surgeries and rehabilitation.
- Robotics Education: A common platform for teaching robotics and control systems.